Hey guys, what is up. In this particular post, we will be talking about selection sort, its working, algorithm, and program. Also, about bubble sort explanation, algorithm, and its program.
Updated on 29/9/2021
KEY POINTS
NOTE:
Sorting can be used in searching to arrange the elements in ascending and descending order. It is mostly used in the binary search to arrange elements in a sorted manner.
Selection Sort:
Selection sort is a mixture of sorting and searching of an element in an array. In this, the loop works for n minus one times. The main aim of this sorting is to first find the smallest element and place it in its desired position. It is done with the help of swapping. Swapping takes place with the use of the third element. The first element at zero position is compared with all the other elements. When the smallest element is found then swapping is done. And then element which is to be placed at the second position is found and so on.
ARRAY | MOVE 1 | MOVE 2 | MOVE 3 | MOVE 4 |
A[0] = 12 | 2 | 2 | 2 | 2 |
A[1] = 6 | 6 | 5 | 5 | 5 |
A[2] = 9 | 9 | 9 | 6 | 6 |
A[3] = 2 | 12 | 12 | 12 | 9 |
A[4] = 5 | 5 | 6 | 9 | 12 |
Selection Sort Algorithm:
a[ ] is an array, where n is the size of array, i,j,n, third are initialized
Step 1: i=0
Step 2: Repeat steps 3 to 7 while i < n-1
Stair 3: j=i+1
Step 4: Repeat steps 5 and 6 while j < n
Step 5: if a[ j ] < a[i]
third= a[ j ], a[j] = a[i], a[i] = third
Stair 6: j=j+1
{ End of inner loop }
Step 7: i=i+1
{ End of outer loop }
Step 8: Stop
Program:
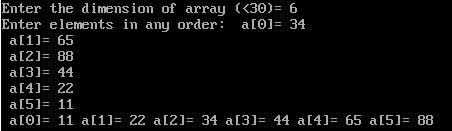
#include <iostream.h>
#include <conio.h>
int main()
{
clrscr();
const max=30;
int a[max], n, i, j, temp;
cout<<“Enter the dimension of array (<30)= “;
cin>>n;
cout<<“Enter elements in any order: “;
for (i=0; i<n; i++)
{
cout<<” a[“<<i<<“]= “;
cin>>a[i];
}
i=0;
while ( i< n-1)
{
j=i+1;
while( j<n )
{
if ( a[j] < a[i])
{
//swapping of elements takes place
temp= a[j];
a[j]=a[i];
a[i]=temp;
}
j=j+1;
}
i=i+1;
}
for (i=0; i<n; i++)
{
cout<<” a[“<<i<<“]= “;
cout<<a[i];
}
return 0;
}
Bubble Sort:
This type of sorting works on the principle of continuous swapping. This swapping takes place between two adjacent elements. If the second element is smaller than the first element then swapping takes place. If the second element is larger than the first element then swapping is not done. This sorting is not used for large data sets. It takes a lot of memory and time.
ARRAY | STAIR 1 | STAIR 2 | STAIR 3 | STAIR 4 |
A[0] = 12 | 6 | 6 | 1 | 1 |
A[1] = 6 | 9 | 1 | 5 | 5 |
A[2] = 9 | 1 | 5 | 6 | 6 |
A[3] = 1 | 5 | 9 | 9 | 9 |
A[4] = 5 | 12 | 12 | 12 | 12 |
Bubble sort algorithm:
a[ ] is an array, where n is the size of array, i,j,n, temp are initialized
Step 1: i=0
Step 2: Repeat steps 3 to 7 while i < n-1
Stair 3: j=i+1
Step 4: Repeat steps 5 and 6 while j < n-i-1
Step 5: if a[ j ] < a[j+1]
temp= a[j], a[j] = a[j+1], a[j+1] = temp
Stair 6: j=j+1
{ End of inner loop }
Step 7: i=i+1
{ End of outer loop }
Step 8: Stop
Program:

#include <iostream.h>
#include <conio.h>
int main()
{
clrscr();
const max=30;
int a[max], n, i, j, temp;
cout<<“Enter the dimension of array (<30)= “;
cin>>n;
cout<<“Enter elements in any order: “;
for (i=0; i<n; i++)
{
cout<<” a[“<<i<<“]= “;
cin>>a[i];
}
i=0;
while ( i< n-1)
{
j=0;
while( j< n-i-1 )
{
if ( a[j] > a[j+1])
{
temp= a[j];
a[j]=a[j+1];
a[j+1]=temp;
}
j=j+1;
}
i=i+1;
}
i=0;
cout<<“Sorted array”<<endl;
for (i=0; i<n; i++)
{
cout<<” a[“<<i<<“]= “;
cout<<a[i];
}
return 0;
}
In the upcoming post, you will be getting information related to Insertion sort and crypto-currency or about the working of the sensors. If you haven’t read our previous post on binary and linear algorithms and programs then go and read this. Also, stay tuned for the upcoming post.
Nice post